مقدمه
در این جلسه، بهزاد فرهادی مفاهیم کلیدی جاوا اسکریپت مرتبط با آرایهها و حلقه فور (for) را معرفی میکند. او نحوه تعریف و استفاده از آرایهها را توضیح داده و تفاوت ایندکسگذاری در انسانها و کامپیوتر را بررسی میکند. همچنین، با یک مثال محاسبه حاصل جمع اعداد از ۱ تا ۵۰، پیچیدگی زمانی دو روش مختلف (حلقه for
و فرمول ریاضی) را مقایسه میکند.
موضوعات اصلی
۱. معرفی آرایهها
آرایهها به عنوان راهی برای ذخیره چندین مقدار تحت یک نام متغیر معرفی میشوند.
به جای تعریف جداگانه متغیرها مانند:
let fruit1 = "سیب";
let fruit2 = "موز";
let fruit3 = "پرتقال";
میتوان از آرایه استفاده کرد:
let fruits = ["سیب", "موز", "پرتقال"];
این روش دسترسی و خوانایی کد را بهبود میبخشد.
۲. درک ایندکسگذاری در آرایهها
بر خلاف انسانها که شمارش را از ۱ شروع میکنند، کامپیوتر از ۰ آغاز میکند.
مثال:
fruits[0]
→"سیب"
fruits[1]
→"موز"
fruits[2]
→"پرتقال"
برای چاپ این مقادیر از
console.log()
استفاده میشود.
۳. مثال عملی: چاپ نامهای کاربری
به جای تعریف جداگانه متغیرها:
let user1 = "احمد";
let user2 = "بهزاد";
let user3 = "علی";
این روش امکان دسترسی راحتتر و سریعتر به دادهها را فراهم میکند.
۴. استفاده از حلقه for
برای کار با دادهها
به جای چاپ دستی هر مقدار:
console.log(users[0]);
console.log(users[1]);
console.log(users[2]);
از یک حلقه
for
استفاده میکنیم:
for (let i = 0; i < users.length; i++) {
console.log(users[i]);
}
این روش مقیاسپذیر بوده و برای هزاران داده مناسب است.
۵. مسئله محاسبه مجموع: حلقه vs فرمول ریاضی
دو روش برای جمع اعداد ۱ تا ۵۰:
استفاده از حلقه
for
.استفاده از فرمول ریاضی:
S = n(n+1)/2
مقایسه پیچیدگی زمانی:
حلقه
for
: پیچیدگی O(n) (خطی).فرمول ریاضی: پیچیدگی O(1) (ثابت و بسیار سریعتر).
نتیجهگیری
در این جلسه، مفاهیم آرایهها و حلقه for
بررسی شد. مزایای آرایهها نسبت به تعریف متغیرهای جداگانه توضیح داده شد و تفاوت شمارش انسانی و شمارش کامپیوتری برای درک بهتر ایندکسگذاری در آرایهها مورد بحث قرار گرفت. همچنین، با مقایسه حلقه for
و فرمول ریاضی، اهمیت انتخاب روش بهینه و سریعتر در برنامهنویسی مشخص شد.
Summary of “JavaScript by Behzad Farhadi – Session 10, Part 01”
Introduction
In this session, Behzad Farhadi introduces key JavaScript concepts related to arrays and loops, particularly the for
loop. He explains how to define and manipulate arrays efficiently and demonstrates the difference between indexing in human counting versus how computers interpret indices. Additionally, he compares using loops versus mathematical formulas for summation problems, highlighting time complexity differences.
Main Topics Covered
1. Introduction to Arrays
Arrays are introduced as a way to store multiple values under a single variable name.
Instead of defining separate variables for each value (e.g.,
let fruit1 = "Apple"; let fruit2 = "Banana"; let fruit3 = "Orange";
), arrays allow grouping these values together.Example:
let fruits = ["Apple", "Banana", "Orange"];
This approach simplifies access to elements and improves code readability.
2. Understanding Indexing in Arrays
Unlike humans who start counting from 1, computers start indexing from 0.
Example:
fruits[0]
→"Apple"
fruits[1]
→"Banana"
fruits[2]
→"Orange"
Behzad demonstrates how to print these values using
console.log()
.
3. Practical Example: Printing Usernames
Instead of defining usernames individually:
let user1 = "Ahmad";
let user2 = "Behzad";
let user3 = "Ali";
We use an array:
let users = ["Ahmad", "Behzad", "Ali", "Bahman"];
This allows easy iteration and retrieval using indices.
4. Using Loops for Efficient Data Handling
Instead of manually printing each element:
console.log(users[0]);
console.log(users[1]);
console.log(users[2]);
A for
loop automates this:
for (let i = 0; i < users.length; i++) {
console.log(users[i]);
}
his approach is scalable, especially when dealing with thousands of elements.
5. Summation Problem: Loop vs Mathematical Formula
The session demonstrates summing numbers from
1
to50
using:A
for
loop.A direct mathematical formula:
S = n(n+1)/2
Comparing the time complexity:
Loop: O(n)O(n)O(n) complexity (linear time).
Formula: O(1)O(1)O(1) complexity (constant time).
Conclusion
This session covered the fundamentals of arrays and their advantages over individual variable declarations. Behzad also explained how for
loops simplify data handling and demonstrated efficient ways to solve mathematical problems. Understanding indexing differences between humans and computers is crucial for correctly accessing array elements. Finally, comparing loops with mathematical formulas helps in writing more efficient code.
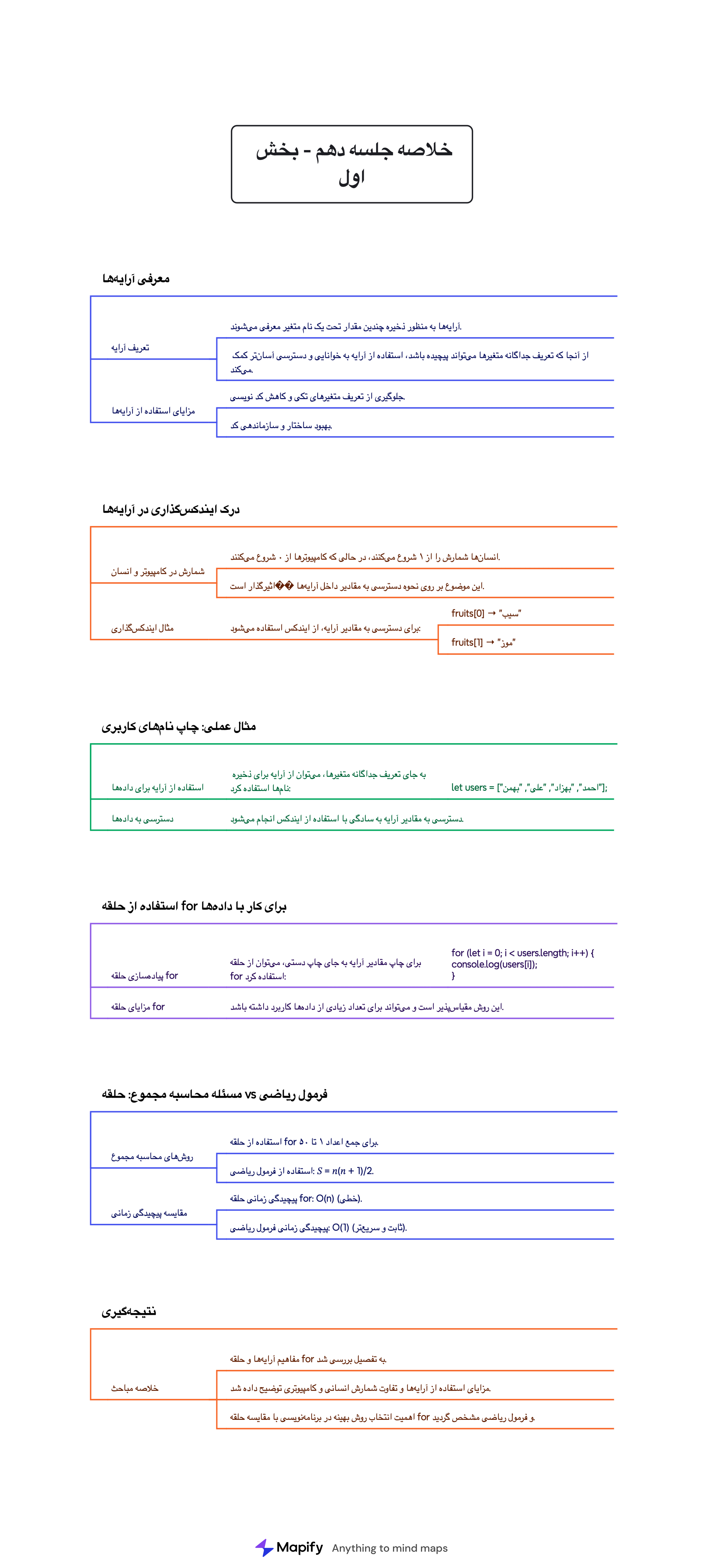