جاوااسکریپت با بهزاد فرهادی |خلاصه جلسه ۱۰ – بخش دوم
مقدمه
در این بخش از آموزش، بهزاد فرهادی به بررسی ساختار حلقهها در زبان جاوااسکریپت میپردازد و به طور خاص حلقه for
را معرفی میکند. هدف این جلسه، آموزش نحوه اجرای کارهای تکراری به شکلی خودکار و بهینه، مثل چاپ یک بازه از اعداد، فیلتر کردن اعداد خاص (مثل اعداد فرد)، و محاسبه مجموع اعداد است.
مفاهیم اصلی
۱. چاپ اعداد ۱ تا ۵۰ با استفاده از حلقه for
در ابتدا اشاره میشود که چاپ اعداد به صورت دستی با
console.log()
وقتگیر است.سپس حلقه
for
معرفی میشود:
for (let index = 1; index <= 50; index++) {
console.log(index);
}
حلقه از ۱ شروع شده، تا ۵۰ ادامه دارد و در هر مرحله ۱ واحد به مقدار افزوده میشود.
در هر بار اجرا، عدد فعلی چاپ میشود.
۲. چاپ فقط اعداد فرد از ۱ تا ۵۰
هدف: فقط اعداد فرد را چاپ کنیم (۱، ۳، ۵، …، ۴۹).
پیشنهاد میشود به جای افزایش یکبهیک،
index
را ۲ تا ۲ تا زیاد کنیم:
for (let index = 1; index <= 50; index += 2) {
console.log(index);
}
اشتباه رایج:
index + 2
که فقط یک عبارت محاسباتی است و تغییری در مقدار
index
ایجاد نمیکند، و در نتیجه حلقه بینهایت میشود.راهحل: استفاده از
index = index + 2
یاindex += 2
.
۳. جلوگیری از حلقههای بینهایت
اگر مقدار جدید به
index
نسبت داده نشود، شرط حلقه هیچگاه نقض نمیشود.مثال اشتباه:
index + 2
مثال صحیح:
index += 2
۴. چاپ اعداد زوج با شروع از صفر
اگر حلقه از صفر شروع شود و گام آن ۲ باشد، اعداد زوج چاپ میشوند.
برای چاپ اعداد فرد، باید از ۱ شروع کرد.
۵. محاسبه مجموع اعداد از ۱ تا ۵۰
یک متغیر
sum
تعریف میشود و با مقدار صفر مقداردهی اولیه میگردد.از حلقه برای جمع کردن مقادیر استفاده میشود:
let sum = 0;
for (let index = 1; index <= 50; index++) {
sum += index;
}
console.log(sum);
خروجی این کد، مجموع تمام اعداد از ۱ تا ۵۰ خواهد بود.
جمعبندی
در این بخش از جلسه ۱۰، بهزاد فرهادی:
نحوه استفاده از حلقه
for
برای اجرای خودکار کدهای تکراری را توضیح داد.چاپ دنبالهای از اعداد، فیلتر اعداد فرد و زوج، و جمع اعداد را آموزش داد.
اشتباهات رایج مثل استفاده اشتباه از
index + 2
را تشریح کرد.و در نهایت، با ارائه مثالهای ساده، پایهای محکم برای یادگیری منطق و تکرار در جاوااسکریپت فراهم کرد.
JavaScript by Behzad Farhadi | Session 10 – Part 02
Introduction
In this session, Behzad Farhadi continues teaching JavaScript fundamentals with a focus on looping structures, particularly the for
loop. The goal is to help beginners understand how to automate repetitive tasks, such as printing a sequence of numbers, filtering specific patterns like odd numbers, and calculating the sum of a range.
Main Concepts and Explanations
1. Printing Numbers from 1 to 50 Using a for
Loop
Initially, Behzad explains how one might manually use
console.log()
to print numbers, but highlights the inefficiency of writing it 50 times.He introduces the
for
loop as a better solution:
for (let index = 1; index <= 50; index++) {
console.log(index);
}
The loop:
Starts at 1 (
let index = 1
)Runs while
index <= 50
Increments
index
by 1 (index++
)
Each iteration logs the current
index
, from 1 to 50.
2. Printing Only Odd Numbers from 1 to 50
The task: print only odd numbers and skip even ones (i.e., 1, 3, 5, …, 49).
He suggests increasing the index by 2 each time:
for (let index = 1; index <= 50; index += 2) {
console.log(index);
}
A common mistake is using:
index + 2
which doesn’t actually update the loop variable. This causes an infinite loop.
Correct syntax is
index = index + 2
orindex += 2
.
3. Avoiding Infinite Loops
If you forget to assign the new value back to
index
, the loop condition never fails.Example of the wrong approach:
index + 2 // This does nothing meaningful to `index`
Corrected using:
index += 2
Printing Even Numbers by Adjusting Start Value
If the loop starts at 0 instead of 1 and increments by 2, it prints even numbers.
To print odd numbers, the loop should start at 1.
5. Calculating the Sum of Numbers from 1 to 50
Introduces a variable
sum
initialized to 0.Uses a loop to add each number to the sum:
let sum = 0;
for (let index = 1; index <= 50; index++) {
sum += index;
}
console.log(sum);
This results in the total sum of all integers from 1 to 50.
Conclusion
In this part of Session 10, Behzad Farhadi:
Demonstrated the power of
for
loops in automating repetitive code.Explained step-by-step how to print sequences of numbers.
Clarified common mistakes (like improper incrementation).
Showed how to manipulate the loop to filter odd/even numbers.
Illustrated how to accumulate values using a loop (e.g., total sum).
By practicing these patterns, learners gain a solid foundation for more advanced logic and iteration in JavaScript.
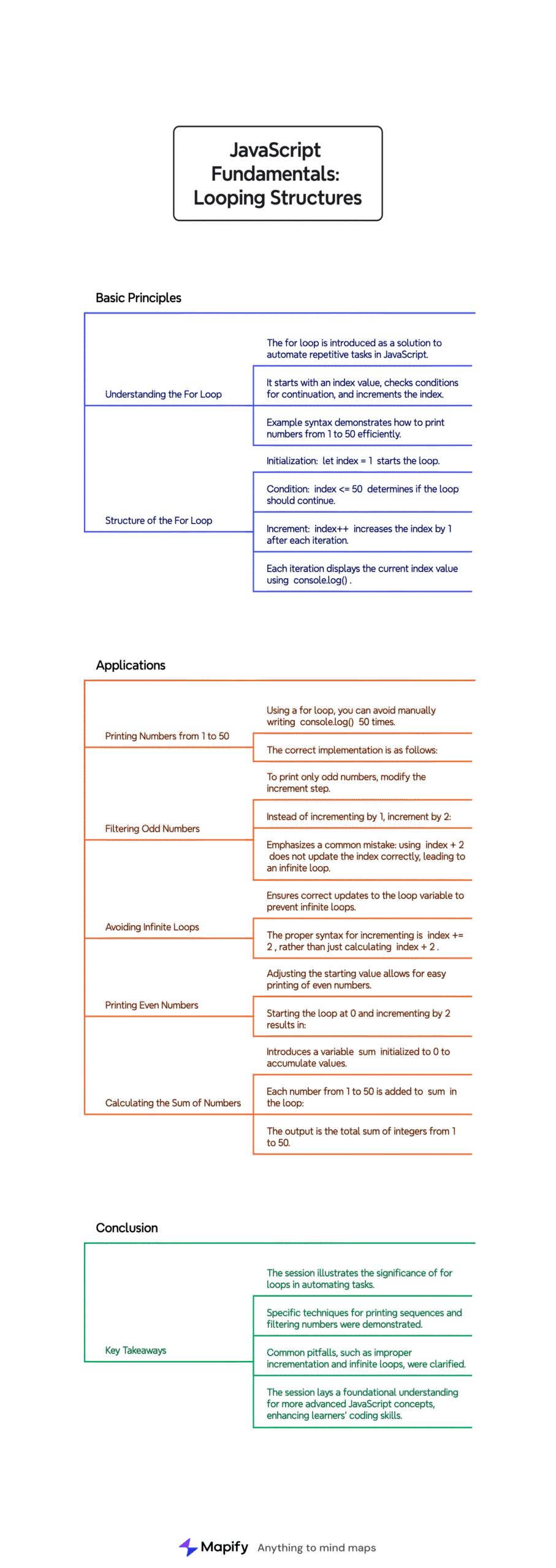