خلاصه جلسه پنجم آموزش جاوا اسکریپت – بهزاد فرهادی
مقدمه
در این جلسه، بهزاد فرهادی چندین مفهوم کلیدی در جاوا اسکریپت را معرفی میکند. مباحث مطرحشده شامل موارد زیر هستند:
- عملگرهای انتصاب ترکیبی
- عملگر باقیمانده
- عملگرهای افزایش و کاهش
- تفاوت بین
var
،let
وconst
در تعریف متغیرها - کاراکترهای خاص در رشتهها
- الحاق رشتهها
این مفاهیم با مثالهایی توضیح داده شده و در کنسول مرورگر نمایش داده میشوند.
مباحث اصلی
۱. تعریف متغیرها: var
، let
و const
var
- متغیرهای تعریفشده با
var
قابل تغییر و بازتعریف در یک حوزه (Scope) هستند. - این ویژگی میتواند در پروژههای بزرگ باعث خطاهای ناخواسته شود.
- مثال:
var cat = "Meow";
var cat = "Woof"; // بدون خطا، اما ممکن است رفتار غیرمنتظره ایجاد کند
console.log(cat); // خروجی: "Woof"
let
- متغیرهای
let
فقط یکبار در یک محدوده تعریف میشوند اما مقدارشان قابل تغییر است. - این ویژگی از بازتعریف ناخواسته متغیرها جلوگیری میکند.
- مثال:
let cat = "Meow";
let cat = "Woof"; // خطا: 'cat' قبلاً تعریف شده است
const
const
برای مقادیری استفاده میشود که نباید تغییر کنند.- تلاش برای تغییر مقدار یک
const
باعث خطا میشود. - مثال:
const PI = 3.14;
PI = 3.1415; // خطا: مقدار متغیر ثابت تغییر نمیکند
۲. عملگرهای افزایش (++
) و کاهش (--
)
++
مقدار متغیر را یک واحد افزایش میدهد.--
مقدار متغیر را یک واحد کاهش میدهد.- مثال:
let num = 7;
num++; // معادل num = num + 1
console.log(num); // خروجی: 8
num--; // معادل num = num - 1
console.log(num); // خروجی: 7
۳. عملگر باقیمانده (%
)
- برای محاسبه باقیمانده تقسیم دو عدد استفاده میشود.
- مثال:
console.log(10 % 4); // خروجی: 2
console.log(13 % 2); // خروجی: 1 (عدد فرد)
console.log(14 % 2); // خروجی: 0 (عدد زوج)
۴. کاراکترهای خاص در رشتهها
- برخی از کاراکترهای خاص در رشتهها:
\n
→ رفتن به خط جدید\t
→ تب\'
→ علامت نقلقول تکی\"
→ علامت نقلقول دوتایی\\
→ بکاسلش
- مثال:
console.log("Hello\nWorld");
// خروجی:
// Hello
// World
۵. الحاق رشتهها
- برای اتصال دو رشته از
+
استفاده میشود. - مثال:
let firstName = "Behzad";
let lastName = "Farhadi";
let fullName = firstName + " " + lastName;
console.log(fullName); // خروجی: "Behzad Farhadi"
نتیجهگیری
این جلسه به مرور عملگرهای مهم و نحوه تعریف متغیرها در جاوا اسکریپت پرداخت. بهزاد بر استفاده از let
به جای var
و استفاده از const
برای مقادیر ثابت تأکید کرد. همچنین، کاراکترهای خاص در رشتهها و روشهای الحاق رشتهها بررسی شدند، که به درک بهتر نحوه مدیریت دادهها در جاوا اسکریپت کمک میکند.
Summary of JavaScript Session 5 – Behzad Farhadi
Introduction
In this session, Behzad Farhadi introduces several key JavaScript concepts. The lesson covers:
- Compound assignment operators
- The remainder operator
- Increment and decrement operators
- Variable declarations using
var
,let
, andconst
- Special characters in strings
- String concatenation
These concepts are explained with examples and demonstrated in the browser console.
Main Topics Covered
1. Variable Declarations: var
, let
, and const
var
- Variables declared with
var
can be reassigned and redeclared within the same scope. - This can cause issues in large projects where unintended redeclaration might lead to errors.
- Example:
var cat = "Meow";
var cat = "Woof"; // No error, but may cause unexpected behavior
console.log(cat); // Outputs: "Woof"
let
let
variables can be reassigned but not redeclared within the same scope.- This helps prevent accidental overwriting of variables.
- Example:
let cat = "Meow";
let cat = "Woof"; // Error: Identifier 'cat' has already been declared
const
const
is used for defining values that should not change.- Trying to reassign a
const
variable results in an error. - Example:
const PI = 3.14;
PI = 3.1415; // Error: Assignment to constant variable
2. Increment (++
) and Decrement (--
) Operators
++
increases the value by 1.--
decreases the value by 1.- Example:
let num = 7;
num++; // Equivalent to num = num + 1
console.log(num); // Outputs: 8
num--; // Equivalent to num = num - 1
console.log(num); // Outputs: 7
3. Remainder Operator (%
)
- Used to find the remainder when dividing two numbers.
- Example:
console.log(10 % 4); // Outputs: 2
console.log(13 % 2); // Outputs: 1 (Odd number)
console.log(14 % 2); // Outputs: 0 (Even number)
4. Special Characters in Strings
- Some special characters in strings:
\n
→ Newline\t
→ Tab\'
→ Single quote\"
→ Double quote\\
→ Backslash
- Example:
console.log("Hello\nWorld");
// Outputs:
// Hello
// World
5. String Concatenation
- Strings can be concatenated using
+
. - Example:
let firstName = "Behzad";
let lastName = "Farhadi";
let fullName = firstName + " " + lastName;
console.log(fullName); // Outputs: "Behzad Farhadi"
Conclusion
This session provided an overview of essential JavaScript operators and variable declarations. Behzad emphasized best practices, such as using let
instead of var
and const
for immutable values. The session also covered special characters and string concatenation, helping learners better understand how JavaScript handles variables and operations.
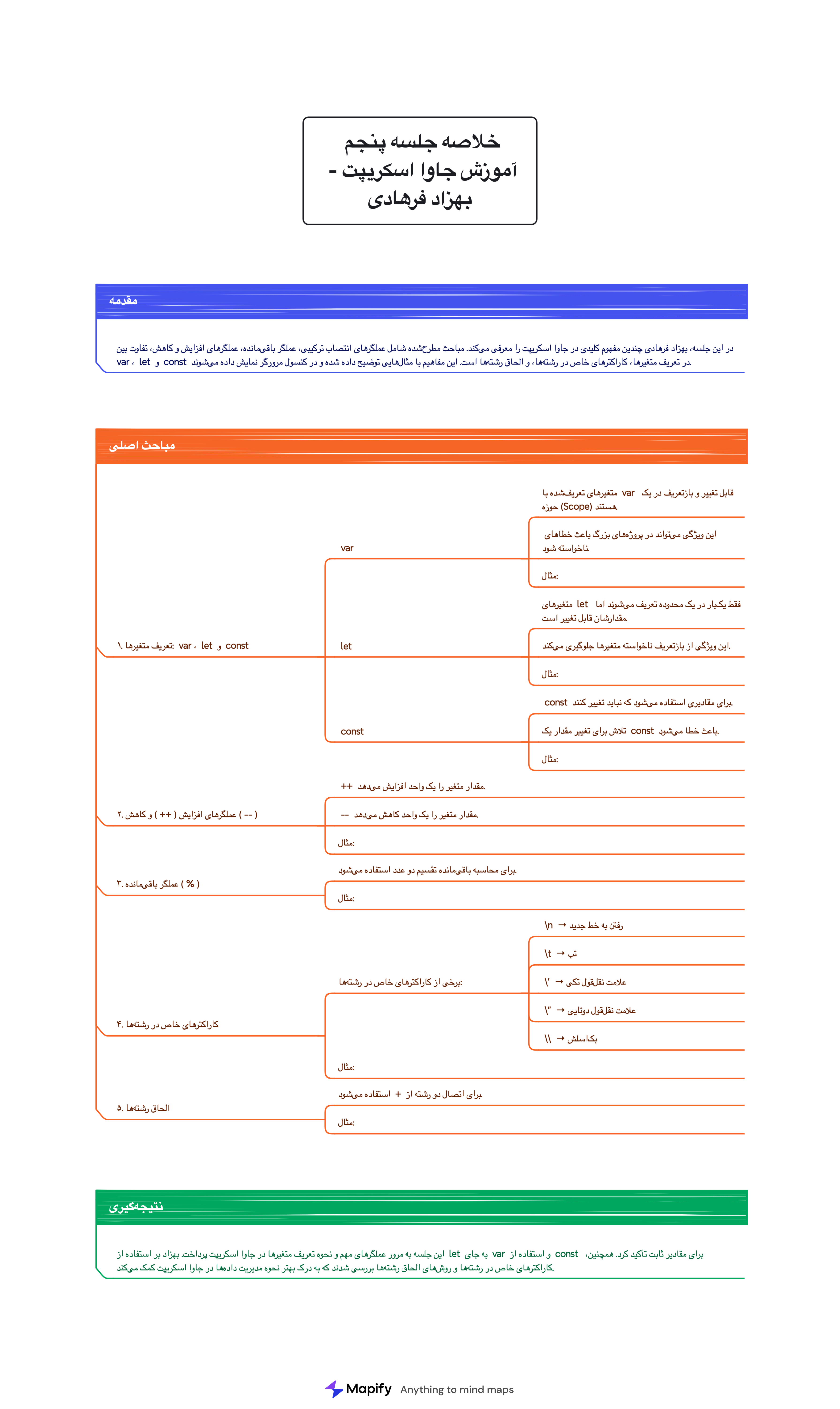